Working with Lists in Python
A Guide to Manipulating Lists in Python
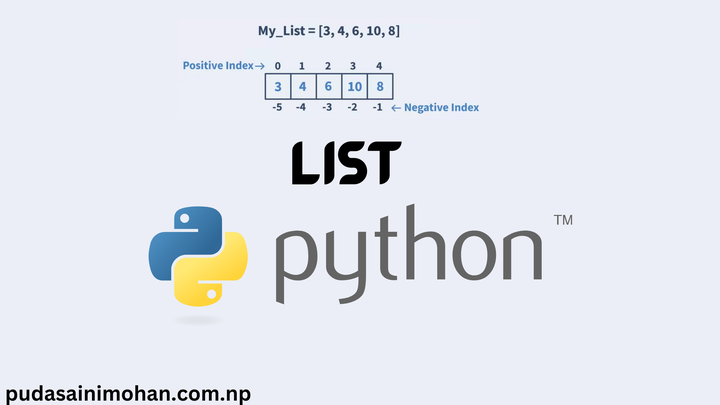
A list in Python is a collection of items, which can be of any data type, including numbers, strings, and other objects. Lists are defined using square brackets [] and the items are separated by commas. For example:
fruits = ["apple", "banana", "cherry"]
numbers = [1, 2, 3, 4, 5]
my_list = ['Ram',23,100.232,'Book',True]
Just like strings, the len()
function will tell you how many items are in the sequence of the list.
len(my_list)
5
Indexing and Slicing
Indexing and slicing are two important concepts in Python when it comes to working with lists.
Indexing refers to accessing individual elements of a list. The elements of a list are stored in a specific order and can be retrieved using their index, which is an integer that starts from 0. For example, consider the following list
my_list = ['Ram',23,100.232,'Book',True]
You can access the first element of the list using the following syntax:
my_list[0]
'Ram'
Slicing, on the other hand, allows you to retrieve a portion of the list. The syntax for slicing is list[start:stop:step]
, where start is the starting index (inclusive), stop is the ending index (exclusive), and step is the increment between elements.
Here’s an example of slicing a list:
my_list[1:]
['Ram', 100.232, True]
here, The expression my_list[1:]
is a slice of the list that starts from the index 1 and goes until the end of the list. In other words, it returns all the elements of the list except for the first one
my_list[::2]
['Ram', 100.232, True]
The expression my_list[::2]
is a slice of the list that starts from the first element (index 0) and goes until the end of the list, taking steps of 2. In other words, it returns every other element of the list, starting from the first one
Concatinating list
You can concatenate two or more lists using the + operator. The + operator creates a new list that is the combination of the elements from the original lists.
my_list + ['new item']
['Ram', 23, 100.232, 'Book', True, 'new item']
Note: This doesn’t actually change the original list!
my_list
['Ram', 23, 100.232, 'Book', True]
You would have to reassign the list to make the change permanent.
my_list = my_list + ['new1']
my_list
['Ram', 23, 100.232, 'Book', True, 'new1']
The *
operator can be utilized for duplication, similar to strings,for example
my_list * 2
['Ram',
23,
100.232,
'Book',
True,
'add new item permanently',
'Ram',
23,
100.232,
'Book',
True,
'add new item permanently']
Basic List Methods
There are several built-in methods in Python that you can use to manipulate lists. Here are some of the most commonly used methods. append(element) - Adds an element to the end of the list
my_list = [1,2,3]
my_list.append(4)
print(list)
[1, 2, 3, 4]
insert(index, element) - Inserts an element at a specific position in the list
my_list.insert(0,"First")
print(my_list)
['First', 1, 2, 3, 4]
remove(element) - Removes the first occurrence of an element from the list.
my_list.remove("First")
print(list)
[1, 2, 3, 4]
pop(index) - Removes the element at a specific position in the list and returns it.
my_list.pop(0)
print(my_list)
[2, 3, 4]
sort() - Sorts the elements of the list in ascending order.
my_list.sort(reverse=True)
print(my_list)
[4, 3, 2]
reverse() - Reverses the order of the elements in the list
my_list.reverse()
print(my_list)
[2, 3, 4]
extend(list) - Adds the elements of one list to the end of another list.
my_list1 =["Book","Copy"]
my_list.append(my_list1)
print(my_list)
[2, 3, 4, ['Book', 'Copy']]
my_list.extend(my_list1)
print(my_list)
[2, 3, 4, ['Book', 'Copy'], 'Book', 'Copy']
The difference between extend() and append() is that the extend method modifies the original list my_list, adding the elements of my_list1 to the end of it. On the other hand, the append() methods creates a new list that contains the elements of both my_list and my_list1.
Nesting Lists
The term “nesting lists” refers to creating a list that contains other lists as its elements. This can be useful when you want to group related items together
Let’s see how this works!
Let's make three lists
lst_1=[1,2,3]
lst_2=[4,5,6]
lst_3=[7,8,9]
Make a list of lists to form a combined_list
combined_list = [lst_1,lst_2,lst_3]
combined_list
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
We can again use indexing to access elements, but now there are two levels for the index. The items in the combined_list object, and then the items inside that list!
access first item in combined_list object
combined_list[0]
[1, 2, 3]
access first item of the first item in the combined_list object
matrix[0][0]
1